1. ImageView
Introducir una imagen desde un sitio local
<ImageView
android:layout_width="match_parent"
android:layout_height="500dp"
android:src="@drawable/flash"
/>
2. ImageView. Cargando imágenes desde una URL
Gradle (Module)
dependencies {
implementation("androidx.core:core-ktx:1.12.0") implementation("androidx.appcompat:appcompat:1.6.1") implementation("com.google.android.material:material:1.10.0") implementation("androidx.constraintlayout:constraintlayout:2.1.4")
implementation("com.squareup.picasso:picasso:2.8")
implementation ("com.github.bumptech.glide:glide:4.16.0")
testImplementation("junit:junit:4.13.2") androidTestImplementation("androidx.test.ext:junit:1.1.5") androidTestImplementation("androidx.test.espresso:espresso-core:3.5.1")
}
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.INTERNET"/>
<application
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView"
android:layout_width="200dp"
android:layout_height="200dp" />
<ImageView
android:id="@+id/imageView2"
android:layout_width="200dp"
android:layout_height="200dp" />
</LinearLayout>
val imageView = findViewById<ImageView>(R.id.imageView)
val imageView2 = findViewById<ImageView>(R.id.imageView2)
Picasso.get().load("https://javigomez.org/ESIC/579.jpg")
.placeholder(R.drawable.ic_launcher_foreground)
.error(R.drawable.ic_launcher_background)
.into(imgSuper)
Glide.with(this)
.load("https://javigomez.org/SOT/img/444.jpg")
.error(R.drawable.ic_launcher_foreground)
.placeholder(R.drawable.ic_launcher_background)
.into(imageView2)
3. ShapeableImageView. Personalizar los marcos de las imágenes
style_image.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<style name="Circular">
<item name="cornerSize">50%</item>
</style>
<style name="RoundedSquare">
<item name="cornerSize">20%</item>
</style>
<style name="CornetCut">
<item name="cornerSize">15dp</item>
<item name="cornerFamily">cut</item>
</style>
<style name="DiamondCut">
<item name="cornerSize">75dp</item>
<item name="cornerFamily">cut</item>
</style>
<style name="SpedificCornerCut">
<item name="cornerSizeTopRight">75dp</item>
<item name="cornerFamilyTopRight">cut</item>
<item name="cornerSizeBottomLeft">75dp</item>
<item name="cornerFamilyBottomLeft">cut</item>
</style>
<style name="SpedificCornerRounded">
<item name="cornerSizeTopRight">75dp</item>
<item name="cornerFamilyTopRight">rounded</item>
<item name="cornerSizeBottomLeft">75dp</item>
<item name="cornerFamilyBottomLeft">rounded</item>
</style>
</resources>
<com.google.android.material.imageview.ShapeableImageView
android:id="@+id/sivCircular"
android:layout_width="150dp"
android:layout_height="150dp"
android:layout_margin="8dp"
android:src="@drawable/flash"
app:shapeAppearanceOverlay="@style/Circular“
app:strokeColor="@color/green"
app:strokeWidth="5dp"
/>

4. WebView
<WebView
android:id="@+id/webView"
android:layout_width="match_parent"
android:layout_height="1500dp"
tools:ignore="WebViewLayout"
/>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.INTERNET"/>
var webView = findViewById<WebView>(R.id.webView)
//Esto es necesario para habilitar js en el navegador
//y poner esta ventana como navegador por defecto
var webSettings: WebSettings=webView.getSettings()
webSettings.javaScriptEnabled=true
webView.setWebViewClient(WebViewClient())
webView.loadUrl("https://javigomez.org")
5. VideoView
<VideoView
android:id="@+id/vvLocal"
android:layout_width="match_parent"
android:layout_height="250dp"
/>
<VideoView
android:id="@+id/vvWeb"
android:layout_width="match_parent"
android:layout_height="250dp"
/>
Creamos un directorio para guardar los videos en local. Los llamamos raw
Luego abrimos el directorio de raw e incorporamos el archivo de video
var vvLocal = findViewById<VideoView>(R.id.vvLocal)
var mcLocal = MediaController(this)
mcLocal.setAnchorView(vvLocal)
var path = "android.resource://" + packageName + "/" + R.raw.movie
vvLocal.setVideoURI(Uri.parse(path))
vvLocal.setMediaController(mcLocal)
vvLocal.start() //Inicia automáticamente
var vvWeb = findViewById<VideoView>(R.id.vvWeb)
var mcWeb = MediaController(this)
mcWeb.setAnchorView(vvWeb)
vvWeb.setVideoPath("https://javigomez.org/ESIC/multi/movie.mp4")
vvWeb.setMediaController(mcWeb)
6. Calendar
<CalendarView
android:id="@+id/cvEjemplo"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/tvFecha"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:text="Fecha seleccionada"
/>
var cvEjemplo = findViewById<CalendarView>(R.id.cvEjemplo)
var tvFecha= findViewById<TextView>(R.id.tvFecha)
cvEjemplo.setOnDateChangeListener { cv,year,month,day ->
var date = "$day/${month+1}/$year"
tvFecha.text="Fecha seleccionada: $date"
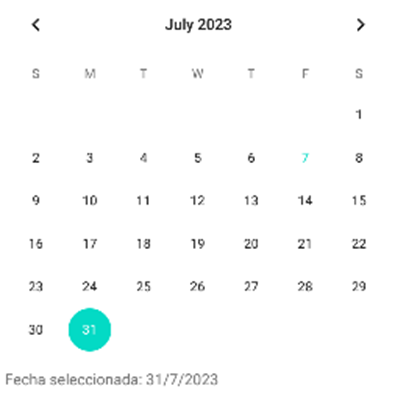
var cvEjemplo = findViewById<CalendarView>(R.id.cvEjemplo)
var tvFecha= findViewById<TextView>(R.id.tvFecha)
cvEjemplo.setOnDateChangeListener { cv,year,month,day ->
var date = "$day/${month+1}/$year"
tvFecha.text="Fecha seleccionada: $date"
}
//Marcar una fecha en el calendario
var calendar = Calendar.getInstance()
calendar.set(2026,5,8)
cvEjemplo.date=calendar.timeInMillis
//empezar por el lunes
cvEjemplo.firstDayOfWeek = Calendar.MONDAY
7. ProgressBarr
ProgressBar circulares
<ProgressBar
android:id="@+id/pbMedium"
android:layout_width="match_parent"
android:layout_height="wrap_content"
style="?android:attr/progressBarStyle"
/>
<ProgressBar
android:id="@+id/pbSmall"
android:layout_width="match_parent"
android:layout_height="wrap_content"
style="?android:attr/progressBarStyleSmall"
/>
<ProgressBar
android:id="@+id/pbLarge"
android:layout_width="match_parent"
android:layout_height="wrap_content"
style="?android:attr/progressBarStyleLarge"
/>
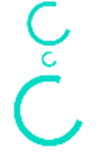
ProgressBar lineales
<ProgressBar
android:id="@+id/pbIndeterminado"
android:layout_width="match_parent"
android:layout_height="wrap_content"
style="?android:attr/progressBarStyleHorizontal"
android:indeterminate="true"
android:indeterminateTint="@color/green"
/>
<ProgressBar
android:id="@+id/pbDeterminado"
android:layout_width="match_parent"
android:layout_height="wrap_content"
style="?android:attr/progressBarStyleHorizontal"
android:max="300"
android:progress="100"
android:progressBackgroundTint="@color/red"
android:progressTint="@color/green"
/>
<ProgressBar
android:id="@+id/pbBarraSecundaria"
android:layout_width="match_parent"
android:layout_height="wrap_content"
style="?android:attr/progressBarStyleHorizontal"
android:max="300"
android:progress="110"
android:progressBackgroundTint="@color/black"
android:progressTint="@color/green"
android:secondaryProgress="130"
android:secondaryProgressTint="@color/red"
/>
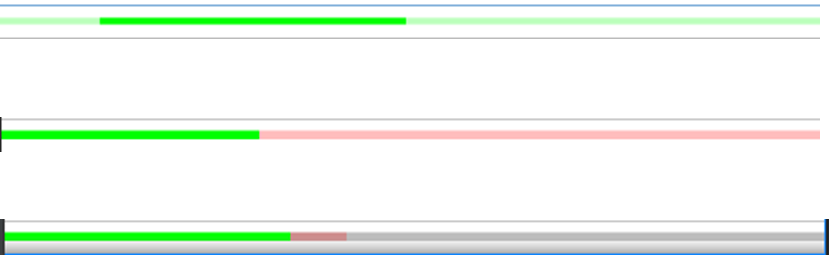
ProgressBar desde código
Se puede establecer los valores iniciales de un ProgressBar en el evento OnCreate de la Activity, pero no se puede mostrar como avanza dentro de este evento. Para ver el avance será necesario crear una corrutina. Para hacer una corrutina será necesario especificar en el gradle
dependencies {
implementation 'androidx.core:core-ktx:1.7.0'
implementation 'androidx.appcompat:appcompat:1.6.1'
implementation 'com.google.android.material:material:1.8.0'
implementation 'androidx.constraintlayout:constraintlayout:2.1.4'
testImplementation 'junit:junit:4.13.2'
androidTestImplementation 'androidx.test.ext:junit:1.1.5'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.5.1'
implementation("org.jetbrains.kotlinx:kotlinx-coroutines-core:1.4.2")
}
var pbDeterminado = findViewById<ProgressBar>(R.id.pbDeterminado)
var pbSecundaria = findViewById<ProgressBar>(R.id.pbSecundaria)
pbDeterminado.max=300
pbDeterminado.progress=0
pbSecundaria.max=300
pbSecundaria.progress=0
pbSecundaria.secondaryProgress=0
GlobalScope.launch {
progressManager(pbDeterminado)
progressManager(pbSecundaria)
}
}
private fun progressManager(pb:ProgressBar){
while (pb.progress < pb.max){
//pb.progress+=5
pb.incrementProgressBy(5)
if (pb.id == R.id.pbSecundaria) pb.incrementSecondaryProgressBy(10)
sleep(100L)
}
}
8. SeekBar
SeekBar es un componente de interfaz de usuario en Android que permite a los usuarios seleccionar un valor dentro de un rango específico, deslizando un indicador a lo largo de una barra
<SeekBar
android:id="@+id/seekBar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:max="100"
android:progress="50"
android:progressTint="@color/green"
android:progressBackgroundTint="@color/red"
android:thumbTint="@color/red"
/>
sb_thumb.xml
<?xml version="1.0" encoding="utf-8"?>
<shape
xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle"
android:tint="@color/purple_200">
<size
android:width="32dp"
android:height="32dp" />
</shape>
sb_progress_bg.xml
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@android:id/background"
android:gravity="center_vertical|fill_horizontal">
<shape android:shape="rectangle"
android:tint="@color/blue">
<corners android:radius="8dp"/>
<size android:height="20dp" />
</shape>
</item>
<item android:id="@android:id/progress"
android:gravity="center_vertical|fill_horizontal">
<scale android:scaleWidth="100%">
<selector>
<item android:state_enabled="false"
android:drawable="@android:color/transparent" />
<item>
<shape android:shape="rectangle"
android:tint="@color/red">
<corners android:radius="8dp"/>
<size android:height="24dp" />
</shape>
</item>
</selector>
</scale>
</item>
</layer-list>
<SeekBar
android:id="@+id/sbCustom"
android:layout_marginTop="30dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:progress="30"
android:thumb="@drawable/sb_thumb"
android:progressDrawable="@drawable/sb_progress_bg"
/>

<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<SeekBar
android:id="@+id/sbCustom"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:max="100"
android:progress="20"
android:progressTint="@color/green"
android:progressBackgroundTint="@color/red"
android:thumbTint="@color/red"
/>
<TextView
android:id="@+id/tvValor"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:text="Valor:"/>
</LinearLayout>
class MainActivity : AppCompatActivity() {
lateinit var sbCustom: SeekBar
lateinit var tvValor: TextView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
sbCustom = findViewById<SeekBar>(R.id.sbCustom)
tvValor = findViewById<TextView>(R.id.tvValor)
sbCustom.setOnSeekBarChangeListener(object : SeekBar.OnSeekBarChangeListener {
override fun onProgressChanged(p0: SeekBar?, p1: Int, p2: Boolean) {
tvValor.setText("Valor: " + p1)
}
override fun onStartTrackingTouch(p0: SeekBar?) {
}
override fun onStopTrackingTouch(p0: SeekBar?) {
}
})
9. RatingBar
RatingBar es un componente de interfaz de usuario en Android que permite a los usuarios seleccionar una calificación o valoración mediante un conjunto de estrellas.
<RatingBar
android:id="@+id/rbEjemplo"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:numStars="5"
android:rating="2.5"
android:progressBackgroundTint="@color/green"
android:progressTint="@color/red"
android:stepSize="0.5"
android:isIndicator="false"
style="@android:style/Widget.Material.Light.RatingBar.Small"
/>
<TextView
android:id="@+id/tvRating"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="3.5"
/>
var rbEjemplo = findViewById<RatingBar>(R.id.rbEjemplo)
var tvRating = findViewById<TextView>(R.id.tvRating)
rbEjemplo.rating = 2.5f
rbEjemplo.setOnRatingBarChangeListener { ratingBar, rating, _ ->
tvRating.text="${rating}/${ratingBar.numStars}"
}
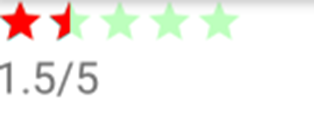
10. SearchView
El SearchView es un componente de interfaz de usuario en Android que permite a los usuarios introducir y buscar texto en una aplicación
searchview_bg.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<corners
android:radius="16dp"/>
<stroke
android:color="#C0BEBE"
android:width="4dp"/>
</shape>
<SearchView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:iconifiedByDefault="false"
android:queryHint="Nombre:"
android:layout_marginTop="30dp"
android:layout_marginHorizontal="16dp"
android:background="@drawable/searchview_bg"
android:queryBackground="@android:color/transparent"
/>
<ListView
android:id="@+id/lvUsers"
android:layout_marginHorizontal="16dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
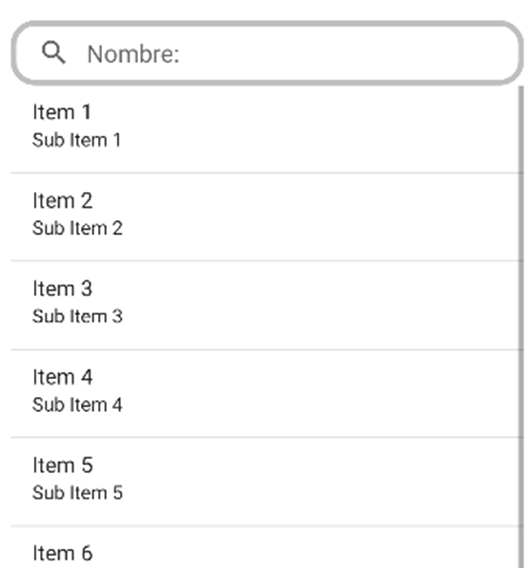
val users=arrayOf("Alberto","Alvaro","Ana","Amparo","Bartolo","Bernardo","Carla","Carlos","Carolina")
val adapterUser: ArrayAdapter<String> = ArrayAdapter(this,android.R.layout.simple_list_item_1,users)
var svUsers=findViewById<SearchView>(R.id.svUsers)
var lvUsers=findViewById<ListView>(R.id.lvUsers)
lvUsers.adapter=adapterUser
svUsers.setOnQueryTextListener(object: SearchView.OnQueryTextListener{
override fun onQueryTextSubmit(query: String?): Boolean {
svUsers.clearFocus()
if (users.contains(query))
adapterUser.filter.filter(query)
return false
}
override fun onQueryTextChange(query: String?): Boolean {
adapterUser.filter.filter(query)
return false
}
<SearchView
android:id="@+id/svUsers"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:iconifiedByDefault="false"
android:queryHint="Nombre:"
android:layout_marginTop="30dp"
android:layout_marginHorizontal="16dp"
android:background="@drawable/searchview_bg"
android:queryBackground="@android:color/transparent"
/>
<ListView
android:id="@+id/lvUsers"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginHorizontal="16dp" />
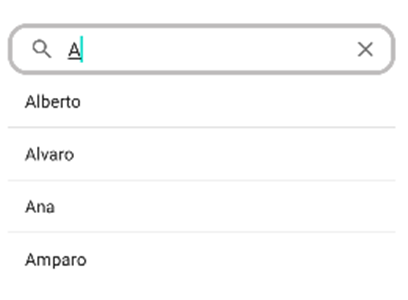
11. Divider
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:text="Texto en la parte superior" />
<View
android:layout_width="wrap_content"
android:layout_height="4dp"
android:background="@color/red"
android:layout_marginHorizontal="10dp"
/>
<View
android:layout_width="4dp"
android:layout_height="100dp"
android:layout_gravity="center"
android:background="@color/green"/>
</LinearLayout>
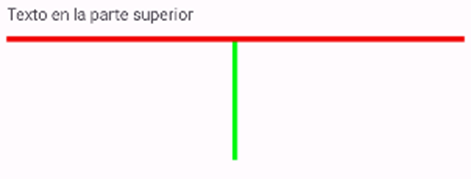